The Ultimate Guide To Smooth Animations In React
Framer Motion has emerged as a powerful library for creating stunning animations in React applications, allowing developers to enhance user experience through smooth transitions and engaging interactions. With the ever-growing demand for visually appealing web applications, mastering Framer Motion can significantly elevate your frontend development skills. In this comprehensive guide, we will delve deep into what Framer Motion is, how it works, and why it has become a go-to solution for animating React components.
In the following sections, we'll explore the fundamental principles of Framer Motion, including its core features, installation process, and practical examples. Additionally, we'll discuss best practices for implementing animations effectively while maintaining optimal performance. Whether you're a seasoned developer or just starting with React, this guide will provide you with valuable insights into harnessing the power of Framer Motion.
As we navigate through the intricacies of this animation library, you'll discover how to create captivating user interfaces that not only look great but also enhance usability. So, let's embark on this journey to master Framer Motion and unlock the full potential of your React applications.
Table of Contents
What is Framer Motion?
Framer Motion is an open-source animation library designed specifically for React. It simplifies the process of creating complex animations and transitions, offering a declarative API that allows developers to define animations in a straightforward manner. With Framer Motion, you can easily animate components, manage gestures, and implement advanced animations with minimal code.
Developed by the team behind Framer, a design tool for interactive prototypes, Framer Motion incorporates advanced animation techniques and principles, making it a versatile choice for developers. Whether you are animating simple elements or creating intricate animations for an entire application, Framer Motion provides the tools you need to achieve stunning results.
Key Benefits of Framer Motion
- Declarative API for easy animation management
- Support for complex animations and transitions
- Integration with React's component lifecycle
- Gesture recognition for interactive animations
- Performance optimization features out of the box
Core Features of Framer Motion
Framer Motion comes packed with features that make it stand out among other animation libraries. Below are some of the core features that developers can leverage:
1. Simple Animations
Framer Motion allows you to create simple animations with just a few lines of code. You can easily animate properties such as opacity, scale, and position by leveraging the `` component.
2. Shared Layout Transitions
This feature enables smooth transitions between different layouts, making it easy to implement animations when components are added or removed from the DOM.
3. Gestures
Framer Motion supports gesture-based animations, allowing developers to trigger animations based on user interactions such as drag, hover, and tap.
4. Layout and Presence
With Framer Motion, you can manage the layout of components and animate their presence in the DOM. This feature enhances user experience by providing visually appealing transitions.
Installation of Framer Motion
Installing Framer Motion is a straightforward process. You can add it to your existing React project using npm or yarn. Below are the commands to install Framer Motion:
npm install framer-motion
yarn add framer-motion
Once installed, you can start using Framer Motion in your React components by importing the relevant modules.
Basic Usage of Framer Motion
To get started with Framer Motion, let's create a simple animation. Below is an example of how to animate a box that scales on hover:
import { motion } from 'framer-motion'; function AnimatedBox() { return ( ); } export default AnimatedBox;
In this example, the box will scale to 1.2 times its original size when hovered over, demonstrating how easy it is to implement animations using Framer Motion.
Advanced Techniques with Framer Motion
After mastering the basics, you can explore advanced techniques to create more complex animations. Here are a few techniques to consider:
1. Custom Animation Variants
Framer Motion allows you to define custom animation variants, enabling you to manage different states of your components easily. You can define the initial, animate, and exit states for your components.
const boxVariants = { hidden: { opacity: 0, scale: 0.8 }, visible: { opacity: 1, scale: 1 }, }; function AnimatedBox() { return ( ); }
2. Staggered Animations
You can create staggered animations for lists of items, which adds a dynamic touch to your UI. By controlling the delay of each item's animation, you can create an engaging effect.
const items = [1, 2, 3, 4]; function StaggeredList() { return ( {items.map((item, index) => ( Item {item} ))} ); }
3. Dragging and Gestures
Framer Motion supports dragging and gestures, allowing you to create interactive components. You can easily implement drag functionality with the `` component.
function DraggableBox() { return ( ); }
Best Practices for Using Framer Motion
To get the most out of Framer Motion, consider the following best practices:
- Keep animations subtle to avoid overwhelming users.
- Use easing functions to create natural motion.
- Optimize performance by limiting the number of animated components.
- Test animations on various devices to ensure a smooth experience.
Performance Optimization in Framer Motion
While Framer Motion is designed for performance, you can further optimize your animations by following these tips:
- Use the `will-change` CSS property to inform the browser about upcoming changes.
- Minimize the use of heavy animations on scroll events.
- Batch animations when possible to reduce reflows and repaints.
Conclusion
Framer Motion is a powerful tool for creating animations in React applications, offering developers the ability to enhance user experience through smooth and engaging transitions. By understanding its core features and best practices, you can leverage Framer Motion to create visually appealing applications that captivate users.
As you continue to explore the capabilities of Framer Motion, don't hesitate to experiment with advanced techniques and optimize your animations for performance. We encourage you to leave a comment below, share this article, or check out other resources to further expand your knowledge on React and animations.
Final Thoughts
Thank you for taking the time to read this guide on Framer Motion. We hope you found it informative and helpful. Be sure to return for more insights and tutorials on web development, where we strive to provide valuable resources for developers of all skill levels.
Also Read
Article Recommendations
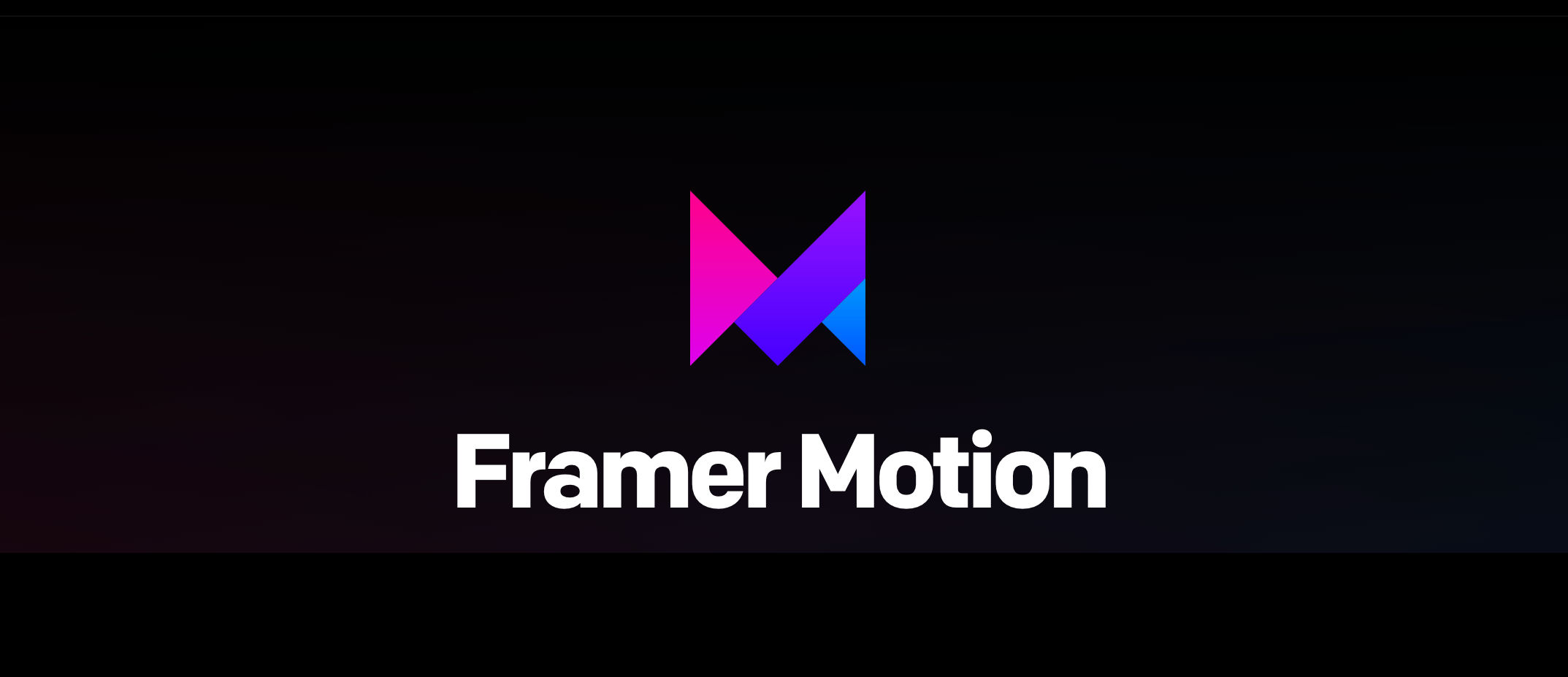
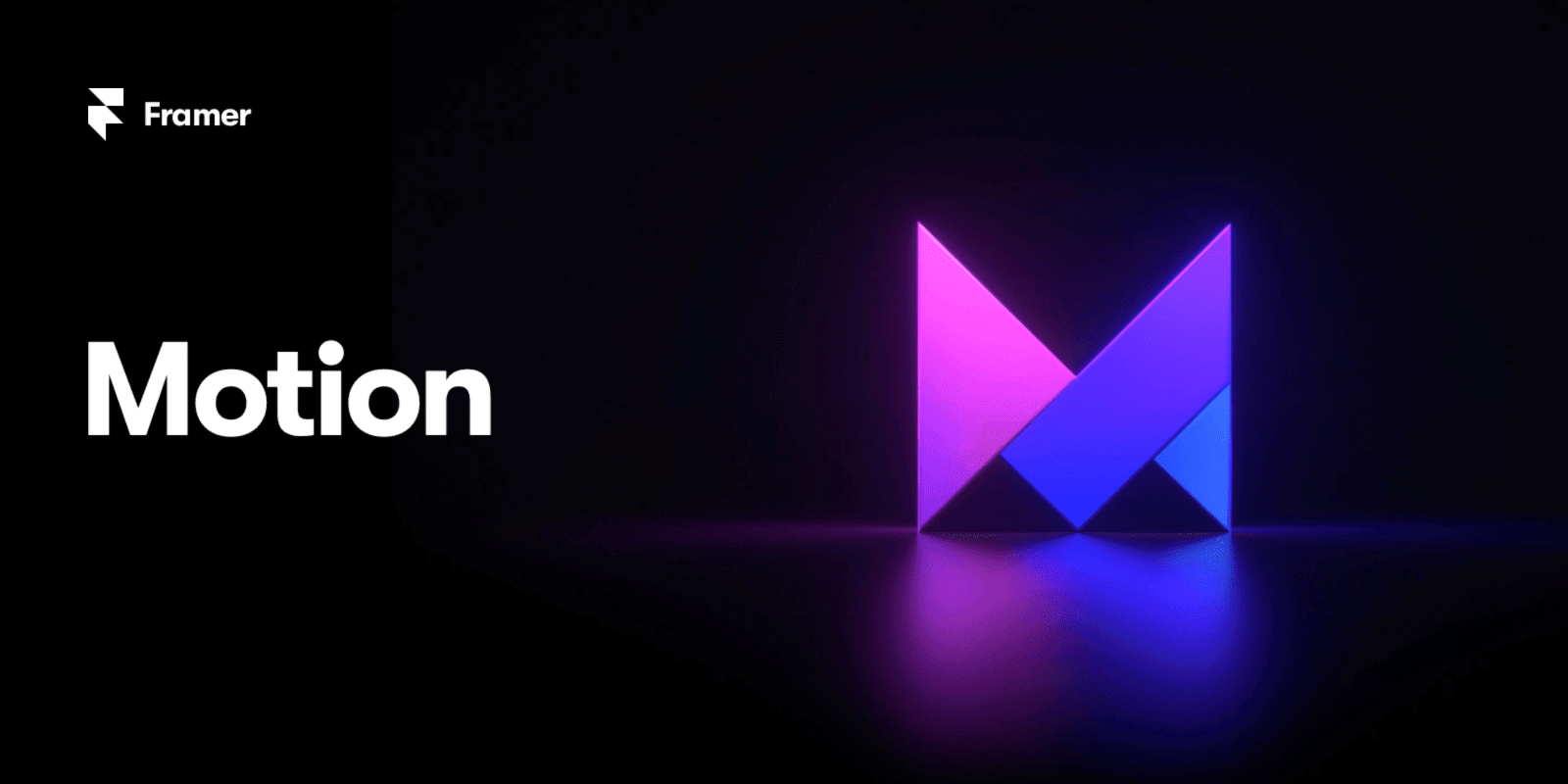
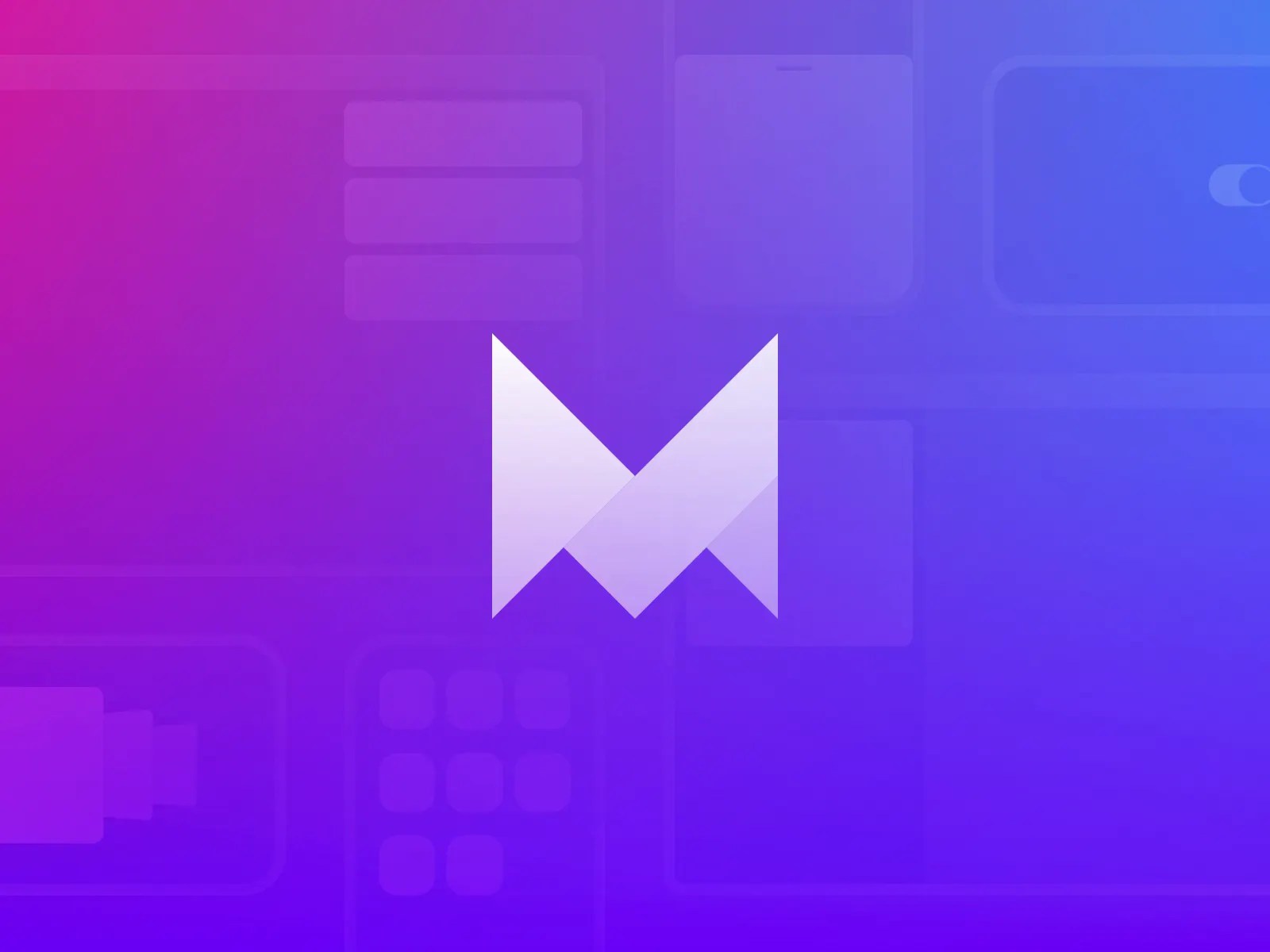
ncG1vNJzZmivp6x7tMHRr6CvmZynsrS71KuanqtemLyue9KtmKtlpJ64tbvKamdonqKWuqa%2BjKamraGfo3upwMyl